Today I'll show you the Android code I used to parse a JSON string from a URL. This code is really useful because nowadays, JSON string is being used by most APIs like Facebook graph API and Google Maps.
You should use JSON in your projects instead of XML because it is lightweight, so much easier to parse, and is supported by most programming language. Recently I wrote about how to generate JSON string with PHP which can be useful for you too.
But if you really have to use XML, you can also take a look at my older post: Parse XML in Android With Three Input Sources
Contents:
1.0 Creating JSON String
Create a JSON string and make it accessible via URL. I created an example for this post, you can see it in this URL: http://demo.codeofaninja.com/tutorials/json-example-with-php/index.php
What it looks like in a JSON viewer:
![]()
As for the code on how to create that JSON string, you can take a look at my older post: Generating JSON String with PHP
What it looks like in a JSON viewer:
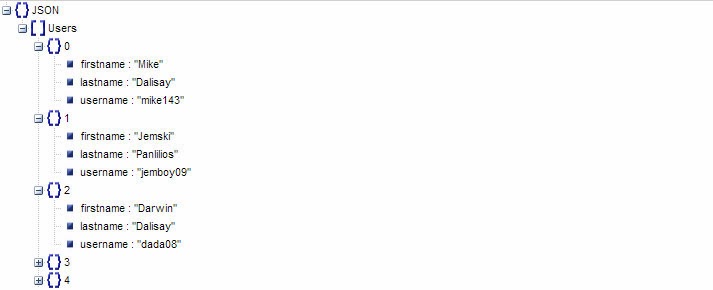
As for the code on how to create that JSON string, you can take a look at my older post: Generating JSON String with PHP
2.0 Create our JSON Parser Class
You can use this JSON parser class with any of your JSON string from URL. Here's our JsonParser.java:
packagecom.example.androidjsonparsing;
importjava.io.BufferedReader;
importjava.io.IOException;
importjava.io.InputStream;
importjava.io.InputStreamReader;
importjava.io.UnsupportedEncodingException;
importorg.apache.http.HttpEntity;
importorg.apache.http.HttpResponse;
importorg.apache.http.client.ClientProtocolException;
importorg.apache.http.client.methods.HttpPost;
importorg.apache.http.impl.client.DefaultHttpClient;
importorg.json.JSONException;
importorg.json.JSONObject;
importandroid.util.Log;
publicclassJsonParser {
finalStringTAG="JsonParser.java";
staticInputStream is =null;
staticJSONObject jObj =null;
staticString json ="";
publicJSONObjectgetJSONFromUrl(Stringurl) {
// make HTTP request
try {
DefaultHttpClient httpClient =newDefaultHttpClient();
HttpPost httpPost =newHttpPost(url);
HttpResponse httpResponse = httpClient.execute(httpPost);
HttpEntity httpEntity = httpResponse.getEntity();
is = httpEntity.getContent();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
try {
BufferedReader reader =newBufferedReader(newInputStreamReader(is, "iso-8859-1"), 8);
StringBuilder sb =newStringBuilder();
String line =null;
while ((line = reader.readLine()) !=null) {
sb.append(line +"\n");
}
is.close();
json = sb.toString();
} catch (Exception e) {
Log.e(TAG, "Error converting result "+ e.toString());
}
// try parse the string to a JSON object
try {
jObj =newJSONObject(json);
} catch (JSONException e) {
Log.e(TAG, "Error parsing data "+ e.toString());
}
// return JSON String
return jObj;
}
}
3.0 Using JSON Parser Class with JSON String
Now we want to make use of our JSON parser with the generated JSON string from URL. But before running our code, make sure you enable internet permission in you AndroidManifest.xml
Now take a look at our MainActivity.java:
<uses-permissionandroid:name="android.permission.INTERNET"></uses-permission>
Now take a look at our MainActivity.java:
packagecom.example.androidjsonparsing;
importorg.json.JSONArray;
importorg.json.JSONException;
importorg.json.JSONObject;
importandroid.os.AsyncTask;
importandroid.os.Bundle;
importandroid.app.Activity;
importandroid.util.Log;
publicclassMainActivityextendsActivity {
@Override
protectedvoidonCreate(BundlesavedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// we will using AsyncTask during parsing
newAsyncTaskParseJson().execute();
}
// you can make this class as another java file so it will be separated from your main activity.
publicclassAsyncTaskParseJsonextendsAsyncTask<String, String, String> {
finalStringTAG="AsyncTaskParseJson.java";
// set your json string url here
String yourJsonStringUrl ="http://demo.codeofaninja.com/tutorials/json-example-with-php/index.php";
// contacts JSONArray
JSONArray dataJsonArr =null;
@Override
protectedvoidonPreExecute() {}
@Override
protectedStringdoInBackground(String... arg0) {
try {
// instantiate our json parser
JsonParser jParser =newJsonParser();
// get json string from url
JSONObject json = jParser.getJSONFromUrl(yourJsonStringUrl);
// get the array of users
dataJsonArr = json.getJSONArray("Users");
// loop through all users
for (int i =0; i < dataJsonArr.length(); i++) {
JSONObject c = dataJsonArr.getJSONObject(i);
// Storing each json item in variable
String firstname = c.getString("firstname");
String lastname = c.getString("lastname");
String username = c.getString("username");
// show the values in our logcat
Log.e(TAG, "firstname: "+ firstname
+", lastname: "+ lastname
+", username: "+ username);
}
} catch (JSONException e) {
e.printStackTrace();
}
returnnull;
}
@Override
protectedvoidonPostExecute(StringstrFromDoInBg) {}
}
}
Thanks for reading this Android JSON parsing tutorial!